Recently, I am enhancing a WordPress child theme based on the official twentyseventeen theme. One modification I want to make is to put a search form in the main navigation, as in the following screenshot.

How could I achieve such a goal?
Yeah, there is an excellent plugin called Ivory Search that can almost put a search form in any location on a WordPress site. However, I’d like to do this by coding.
It turns out pretty easy, once you are familiar with WordPress theme development. Three files need to be modified, functions.php, style.css, and template-parts\navigation\navigation-top.php. Following WordPress best practices, I made these changes in the child theme of twentyseventeen.
First, let’s register a widget sidebar in the functions.php.
function my_widgets_init() {
// Register a sidebar area in the top navigation, for adding search form
register_sidebar(
array(
'name' => __( 'Navigation Sidebar', 'twentyseventeen' ),
'id' => 'sidebar-nav',
'description' => __( 'Add widgets here to appear in top navigation.', 'twentyseventeen' ),
'before_widget' => '<section id="%1$s" class="widget %2$s">',
'after_widget' => '</section>',
'before_title' => '<h2 class="widget-title">',
'after_title' => '</h2>',
)
);
// More initialization settings
}
add_action( 'widgets_init', 'my_widgets_init' );
Then, let’s change the template-parts\navigation\navigation-top.php file, making it look like the following one (for brevity, I ignore all php comment at the file).
<nav id="site-navigation" class="main-navigation" role="navigation" aria-label="<?php esc_attr_e( 'Top Menu', 'twentyseventeen' ); ?>">
<div id="navigation-wrap">
<button class="menu-toggle" aria-controls="top-menu" aria-expanded="false">
<?php
echo twentyseventeen_get_svg( array( 'icon' => 'bars' ) );
echo twentyseventeen_get_svg( array( 'icon' => 'close' ) );
_e( 'Menu', 'twentyseventeen' );
?>
</button>
<?php
wp_nav_menu(
array(
'theme_location' => 'top',
'menu_id' => 'top-menu',
)
);
?>
<?php if ( ( twentyseventeen_is_frontpage() || ( is_home() && is_front_page() ) ) && has_custom_header() ) : ?>
<a href="#content" class="menu-scroll-down"><?php echo twentyseventeen_get_svg( array( 'icon' => 'arrow-right' ) ); ?><span class="screen-reader-text"><?php _e( 'Scroll down to content', 'twentyseventeen' ); ?></span></a>
<?php endif; ?>
</div> <!-- #navigation-wrap -->
<div id="navigation-search">
<?php if (is_active_sidebar("sidebar-nav")):
dynamic_sidebar("sidebar-nav");
endif; ?>
</div> <!-- #navigation-search -->
</nav><!-- #site-navigation -->
Now you can drag any widget into this area in the dashboard.
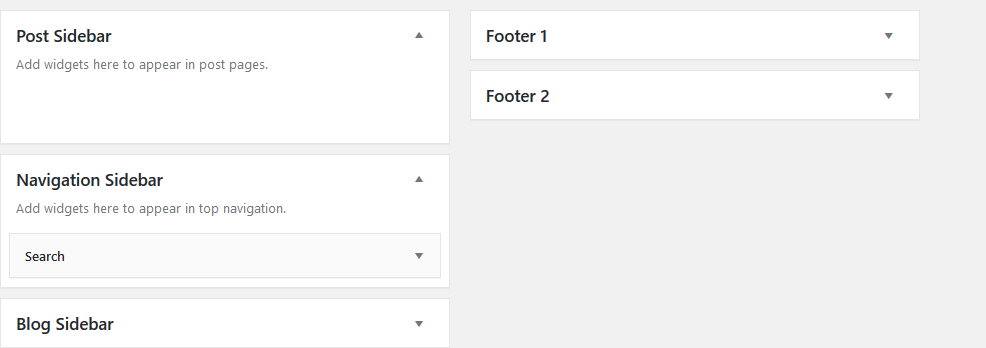
If you want a search form appear here, some styling rules are needed, otherwise the main navigation will look naughty. So put the following CSS rules in the style.css file.
#navigation-wrap {
float: left;
width: 60%;
}
#navigation-search {
width: 30%;
float: right;
margin-right: 0.5em;
}
#navigation-search .widget {
padding: 0;
}
#navigation-search input[type="search"] {
padding: 2px;
}
#navigation-search .icon-search {
height: 18px;
top: -10px;
width: 10px;
}
Done! The search form appears on the main navigation.
There is still an extra styling to do for better responsive layout. I will fix this later.
The code is added to my twentyseventeen child theme which is hosted on Github.